Mastering Python with REST API: Your Essential Guide to Building Robust Web Services
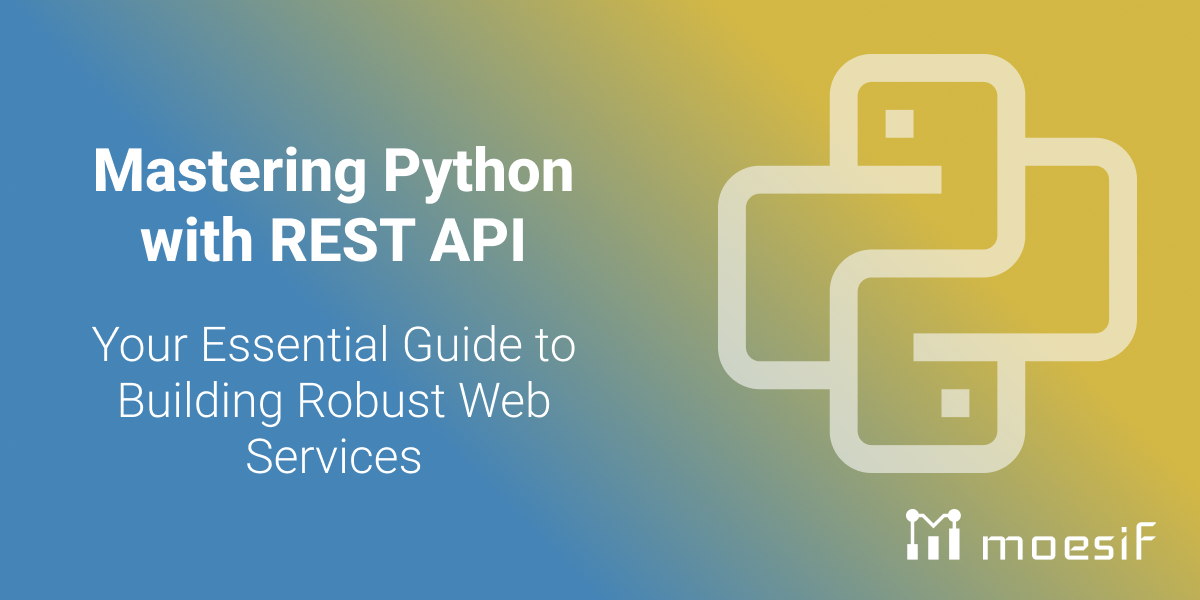
If you’re looking to integrate web services using Python, mastering REST APIs is crucial. This article delivers a clear, step-by-step guide to leveraging Python with REST API’s straightforward syntax and libraries to build and connect with RESTful services. From environment setup to security and beyond, expect practical advice that will elevate your Python with REST API projects seamlessly within the world of REST APIs.
In this guide, we will explore various aspects of REST API development with Python. Starting with the basics of setting up your development environment, we will walk through the process of choosing the right web framework, such as Flask or Django, and configuring your project for success. You will learn how to define and implement API endpoints, manage data serialization, and handle HTTP requests and responses effectively.
Whether you are a seasoned developer or just starting your journey with Python and REST APIs, this guide aims to provide you with the tools and understanding necessary to create powerful, efficient, and secure web services. So, let’s dive in and master the art of building RESTful APIs with Python!
Key Takeaways
- A REST API in Python enables communication over HTTP, allowing data operations in a stateless manner, utilizing frameworks like Flask and Django for development.
- Securing a REST API involves implementing authentication methods like token-based authentication, OAuth, and JSON Web Tokens (JWT), alongside error handling and best security practices.
- API performance and scalability can be enhanced through strategies like caching, filtering, rate limiting, and asynchronous processing, ensuring efficient handling of high traffic and real-time data exchange.
Exploring Python REST API Fundamentals
To master REST APIs, a clear understanding of the foundational elements that constitute this architecture is mandatory. At its core, a REST API is a set of principles that define how web services communicate over HTTP, performing operations on data in a stateless fashion - meaning no client context is stored server-side between requests. With Python at the helm, developers are endowed with a language that’s not only easy to read and write but also inherently geared toward handling web services and making complex data transactions seem effortless.
The allure of Python REST APIs lies in their simplicity and the ability to convey data in formats like JSON, which is both human and machine-readable. This is particularly beneficial when dealing with intricate data structures that require clear and concise communication between the client and the server. Furthermore, the Python community’s contributions to frameworks and libraries make it an even more attractive option for those looking to build their own Python rest APIs and RESTful web services. Access points to these resources, known as API endpoints, are crucial for enabling this communication.
Harnessing the power of Python to develop a REST API means engaging with an ecosystem that’s designed to streamline the development process. This entails a gentle learning curve and a quick transition from concept to execution. The readability of Python code and its compatibility with data interchange formats propels Python to the forefront as a language of choice for creating sophisticated REST APIs that are both efficient and easy to maintain.
Setting Up Your Python Environment for REST API Development
Proper tool assembly is a prerequisite before one fully immerses into REST API development. The journey begins with the installation of Python and its package manager, Pip. This dynamic duo lays the groundwork for managing the libraries and dependencies your project will require. However, the true magic unfolds with the introduction of virtual environments, courtesy of tools like Pipenv. These isolated environments are pivotal in ensuring that dependencies for different projects remain segregated and harmonious.
Once Python and Pip are set up, the subsequent move involves choosing a suitable web framework to structure your REST API. The Python ecosystem is rich with options like Flask, a minimalist framework that provides a sturdy yet flexible foundation for web applications and REST APIs alike. For more comprehensive needs, Django, coupled with its REST framework, offers a robust solution that streamlines the development process, allowing you to focus on crafting quality APIs. A Django REST framework example can demonstrate how this powerful tool can be utilized effectively. Additionally, an API gateway can play a crucial role in managing traffic and providing additional layers of security.
The choices don’t end there; depending on your needs, you might also consider FastAPI, a modern, high-performance framework designed specifically for building APIs with Python 3.6+ types. It’s optimized for speed, making use of Python’s async features to handle large numbers of concurrent clients efficiently. Whichever framework you choose, the goal is to create a development environment that’s both powerful and tailored to your project’s unique requirements.
Installing Python and Virtual Environment Tools
Python interpreter constitutes the bedrock of any Python project. The recommended version is Python 3.6 or newer, which can be acquired from the official Python website. During installation, adding Python to PATH is a crucial step that ensures you can invoke Python from any terminal or command prompt. To confirm a successful installation, a simple command ‘python –version’ or ‘python3 –version’ should display the installed version, signifying that you are ready to embark on your development journey.
After firmly installing Python, the subsequent essential tool is a virtual environment. Virtual environments are isolated spaces where you can install packages and dependencies without affecting the global Python installation. This is where Pipenv shines, providing not only a virtual environment for each project but also managing project-specific dependencies. The result is a neatly organized workspace where projects are insulated from one another, preventing any unwanted clashes between libraries.
To initiate a new project, a simple ‘pipenv shell’ will spawn a fresh virtual environment, while ‘pipenv install’ will start populating it with your chosen packages. As you switch between projects, this workflow ensures that each project’s environment is preserved and that your global Python installation remains pristine. It’s a system that promotes discipline and organization, traits that are invaluable in the fast-paced realm of software development.
Choosing and Installing a Web Framework
Choosing a suitable web framework is equivalent to selecting the ideal tool for a task. Flask, with its minimalistic and extendable nature, offers a lightweight yet powerful platform for developing web applications and REST APIs. It’s particularly suitable for projects where granular control over components is desired. FastAPI, on the other hand, is a more recent addition to the Python web framework scene, built from the ground up with speed, ease of use, and robustness in mind. It is specifically designed for building APIs and boasts features like automatic JSON response generation and data validation with Python-type annotations.
Installing these frameworks is a breeze with pip, Python’s package installer. For Flask, a simple ‘pip install flask’ suffices. FastAPI requires a similar approach, with an additional step of setting up an ASGI server like Uvicorn to handle asynchronous requests, ensuring that your API can keep up with high demand without breaking a sweat.
Each framework comes with its philosophy and set of best practices. Whether you value the simplicity and control of Flask or the modern, feature-rich environment of FastAPI, your choice will set the stage for the development experience that follows. As you install your chosen framework, envision the robust web services you’ll create, services that will soon become integral to the users who rely on them.
Designing Your First RESTful API with Python
Just like every magnificent art piece that originates from a blueprint, RESTful APIs follow the same principle. When designing your first REST API with Python, the focus is on defining clear endpoints that represent the resources your users will interact with. This is where the beauty of REST architecture comes to light - it’s all about simplicity and clarity. With Python’s knack for readable code, you’re empowered to create an API that not only works well but also makes sense to anyone who reads it. Additionally, API versioning is crucial in maintaining backward compatibility as the API evolves.
In RESTful API design, you’ll soon realize that every endpoint you define is a promise of functionality to the client. These endpoints are the access points to the resources your API provides, and they are the very essence of your web service. Crafting these endpoints with thoughtful consideration ensures that your API is intuitive and user-friendly. To achieve this, Python’s frameworks like Flask offer decorators and conventions that help you map out these resources in a way that’s both logical and efficient.
As you lay down the structure of your API, you’re also setting up the rules of engagement for how clients will interact with your web service. These rules are defined by HTTP methods such as:
- GET: for reading resources
- POST: for creating resources
- PUT: for updating resources
- DELETE: for deleting resources
Through this design process, you’re not just building an application programming interface; you’re sculpting an API that will become the backbone of your application’s communication with the outside world.
Defining API Endpoints and Resources
Defining API endpoints and resources is similar to curating a well-structured library catalog, where every book is carefully sorted and readily accessible. In the digital realm of REST APIs, these endpoints are the shelves on which your data - the books - are displayed. Flask empowers you to outline these endpoints elegantly using the @app.route decorator, linking HTTP requests to Python functions that will handle the data interactions.
When it comes to resources, RESTful APIs typically use plural nouns like ‘customers’ or ‘events’, which intuitively represent collections of items or entities. This naming convention speaks volumes about the nature of the data you’re working with, making it clear for anyone who’s navigating your API. A well-thought-out endpoint not only facilitates a smooth user experience but also bolsters the API’s scalability as existing resource and functionalities grow.
As your API matures, the number of endpoints may snowball from a handful to potentially hundreds, each serving a distinct purpose. From listing all resources with the option to filter them, to fetching the details of a specific item, your endpoints will become the road map guiding users through your web service’s resources. With Flask as your guide, you can ensure that this roadmap is not only comprehensive but also intuitive, leading to an API that users can navigate with ease and confidence.
Handling HTTP Requests and Responses
The interaction between a client and server in web applications is orchestrated via HTTP requests and responses. Each HTTP method carries its semantics, with:
- GET for retrieving data
- POST for creating
- PUT and PATCH for updating
- DELETE for removing resources
These methods are the verbs that describe the actions a client wishes to perform via the API.
Response to these requests is just as important, with the incorporated keyword, proper HTTP status code, providing the feedback. They are the nods or shakes of the head from the server, indicating whether a request was successful, if a resource was not found, or if the requested action is not supported. In the headers of these HTTP messages, metadata such as content type and authentication tokens are passed along, adding context to the requests and responses.
In Python, the Requests library offers a streamlined approach to crafting these HTTP messages, allowing developers to focus on the logic of their applications rather than the mechanics of the HTTP protocol. Whether you’re querying data from a REST web service or posting new information, Requests abstracts away the complexities, providing a high-level interface for processing HTTP requests and handling the associated responses.
Implementing REST API Functionality in Python
Once a robust design is established, the next step involves actualizing your REST API. Implementing functionality in Python, particularly using Flask, is a journey of defining endpoints and assigning behaviors to HTTP methods. This process allows us to create a dynamic interface through which clients can retrieve, post, and delete resources at will. To secure access to the API, an API key can be used.
At the heart of this functionality is the request object, through which Flask grants access to incoming data. The request.get_json() method is a simple yet powerful tool that parses incoming JSON payloads, allowing your API to handle the data sent by clients efficiently. Whether you’re adding new resources with a POST request or updating existing ones with PUT, the ability to manage incoming data is crucial.
And what happens when a resource has served its purpose? The DELETE method comes into play, allowing clients to remove resources from the server. In response, the API confirms the action with an empty JSON object, closing the chapter on that particular resource and maintaining the integrity of the web service’s data.
Creating Handlers for HTTP Methods
In the construction of a REST API, handlers for HTTP methods act like individual threads, each symbolizing a discrete action executable on the resources. In Flask, these handlers are functions tied to specific routes, declared with the @app.route decorator, which responds to various HTTP methods like:
- GET for reading data
- POST for creating new entries
- PUT for updating existing ones
- DELETE for removal.
As you map these functions to routes, you’re effectively scripting the interactions your users will have with your API. For instance, a GET request might trigger a function to retrieve and return a list of items in JSON format, using Flask’s jsonify() function to ensure the data is properly formatted.
Conversely, when a POST request hits your API, Flask’s request object springs into action, allowing you to extract the JSON payload and create a new resource based on the data provided.
The PUT and DELETE methods round out the suite of HTTP actions, enabling clients to keep the resources they interact with up-to-date and relevant. Implementing these methods in Flask is straightforward, with functions tailored to process the incoming requests and effect the desired changes on the resources. Whether modifying attributes with PUT or confirming the deletion of an item with DELETE, these handlers ensure that your API remains responsive and efficient.
Working with Data Formats and Serialization
Within the digital ecosystem of a REST API, data formats serve as the communication medium for data. While XML was once prevalent, JSON has risen to prominence for its ease of use and readability, especially in web services. Serialization is the process of converting a data structure into a format that can be easily shared or stored, such as a JSON object. In Python, this process is facilitated by libraries that allow for quick conversion between complex data types and JSON, making it a breeze to send and receive structured data.
Some popular libraries for JSON serialization in Python include:
- json: This is a built-in library in Python that provides functions for encoding and decoding JSON data. To use it, simply import the library in your py file.
- simplejson: This is a third-party library that provides a faster implementation of the json module.
- ujson: This is another third-party library that provides an ultra-fast JSON encoder and decoder for Python.
By using these libraries, you can easily convert Python objects into JSON format and vice versa, allowing for seamless communication with REST APIs.
When a client makes a POST request to your REST API, for example, the data payload is typically included in JSON format. The Content-Type header of the request is set to application/json, signaling to the REST API that the incoming data should be parsed as JSON. If the request body contains a Python dictionary, the requests.post() function will automatically serialize it to a JSON object, making the exchange of data between client and server seamless and efficient.
However, handling web service data isn’t just about the happy path of correct and complete information. An API must also be robust enough to deal with invalid or missing data. In these instances, a well-implemented REST API will return an appropriate status code and a descriptive error message, guiding the client towards rectifying the issue. By managing these edge cases effectively, you ensure a resilient web service that can gracefully navigate the complexities of real-world data exchange.
Securing Your Python REST API
Web services come with their own set of risks, hence, securing your REST API is similar to reinforcing a castle against potential threats. Security is not merely a feature but a foundational aspect of any robust API. From authentication to error handling, there are several layers to consider when shielding your Python REST API from the threats that lurk in the online world.
At the forefront of API security is authentication, the process of verifying the identity of those who wish to access your API’s resources. Implementing authentication mechanisms such as token-based authentication, OAuth, and API keys is not just about keeping out unwanted guests; it’s about ensuring that each request is accompanied by credentials that can be trusted. Storing these keys and tokens securely, separate from your application’s codebase, is a critical practice in maintaining the integrity of your API.
But security doesn’t end with authentication. It extends to the very way your API handles and responds to requests. Providing helpful error messages for failed authentication attempts, correctly implementing HTTPS to encrypt data in transit, and setting up measures for detecting and responding to suspicious activities are all part of the tapestry that makes up a secure REST API. With tools like Flask and the Django REST framework, these security measures can be integrated into your API, establishing a fortified web service that stands strong against the various threats of the digital age.
Authentication Techniques
For safeguarding the integrity of your REST API, authentication techniques act as the primary defense mechanism. Basic authentication, for instance, involves the client sending a username and password with each HTTP request, which the server verifies before allowing access to the requested resources. However, in the vast ocean of the internet, where data breaches are not uncommon, stronger methods are often required. Some stronger authentication methods include:
- Token-based authentication
- OAuth
- JSON Web Tokens (JWT)
- Two-factor authentication (2FA)
- Certificate-based authentication
Two-factor authentication (2FA) adds a layer of security by requiring not just a password and username but also something that only the user has on them, i.e., a piece of information only they should know or have immediately to hand.
Implementing these stronger authentication methods can provide an extra layer of security for your REST API.
Token-based authentication offers a more secure alternative, where encrypted tokens are exchanged between the client and server. These tokens, which are often short-lived, provide a secure way to verify identity without constantly transmitting sensitive information like passwords. FastAPI, with its built-in support for OAuth2 and JWT, makes implementing such robust authentication systems a relatively painless process.
API key authentication is another popular approach, where a unique key is assigned to each client. This key must be included in every request to the API, serving as a stamp of approval that the request is coming from a recognized source. While API keys provide a moderate level of security, they are particularly useful for controlling access and keeping detailed logs of API usage. Securely managing these keys, perhaps with the help of services like Auth0, ensures that your API remains accessible only to those who are authorized.
Error Handling and Security Best Practices
Error handling and security best practices form the next layer of protection, beyond the initial authentication phase. A fortress is only as strong as its weakest point, and in the world of REST APIs, inadequate error handling can be a significant vulnerability. Crafting meaningful error messages that provide clarity without exposing too much information is an art form. It’s about striking a balance between being helpful to legitimate users and giving nothing away to potential attackers.
Password policies, token revocation, and access control lists form the battlements of your API’s defenses.
- Strong password policies and hashed credentials are the sturdy walls that keep intruders at bay.
- Token revocation mechanisms act as the sentries, ready to cut off access at the first sign of compromise.
- Access control lists are the watchtowers, ensuring that each user can only interact with the API resources that they are explicitly allowed to.
But even the most well-defended castle must have a plan for when things go awry. This is where an API wrapper comes in, serving as an isolation layer that can handle access, debugging, and auditing without altering the API itself. Additionally, using the raise_for_status() method from Python’s Requests library helps detect and raise exceptions for HTTP error responses, allowing for swift and decisive error handling.
Enhancing API Performance and Scalability
Performance and scalability are the dual pillars fundamental for creating an API that endures time and demand. As your API grows in popularity and usage, it must be able to handle an increasing number of requests without faltering. This is where strategies such as caching, filtering, and asynchronous processing come into play, ensuring that your REST API can scale gracefully with demand. Additionally, implementing rate limiting can ensure fair usage of API resources, preventing any single user from overwhelming the system.
Caching is like a memory palace, storing frequently access data in a readily available format to reduce the burden on the server. Implementing a read-only caching layer can dramatically decrease latency, making it an essential strategy in environments where the same requests are made repeatedly. Filters, particularly exact match filters, improve performance by narrowing down the data that needs to be parsed and processed, allowing the API to respond more quickly to client requests.
However, there’s more to scalability than just managing traffic. It’s also about the efficient use of resources. Requesting only the necessary fields, disabling event generation for frequently run scripts, and using specific filters in queries are all tactics that reduce overhead and improve the performance of your API. These optimization strategies are essential for maintaining a responsive and reliable service that can keep up with the needs of its users.
Scaling Your API for High Traffic
As user traffic increases, your API should possess the necessary infrastructure to accommodate this surge. Here are some strategies to manage high traffic:
- Load balancers distribute the influx of requests across multiple servers, preventing any single server from becoming overwhelmed.
- A microservices architecture can aid in managing high traffic by breaking down the API into smaller, more manageable services.
- Rate limiting is another crucial mechanism, acting as a regulator to ensure that each user only consumes a fair share of the API’s resources.
Implementing these strategies will help ensure that your API can handle high traffic effectively.
API gateways serve as the harbormasters, directing the flow of requests and responses to and from the API. They not only manage traffic but also offer additional layers of security, analytics, and even monetization opportunities. When combined with asynchronous processing, these strategies enable an API to handle a multitude of requests concurrently, without sacrificing performance. Threading may be suitable for smaller-scale applications, but for those that need to scale, solutions like Celery provide a robust framework for managing asynchronous tasks.
In embracing these scaling strategies, your REST API becomes more than just a tool; it becomes a resilient service capable of weathering the storm of popularity and growth. With the right infrastructure in place, you can ensure that your API remains fast, responsive, and reliable, no matter how many users come knocking.
Asynchronous Processing of HTTP Requests
Today’s web is a dynamic and interactive sphere where users anticipate real-time responses and fluid experiences. Asynchronous processing in REST APIs is the key to fulfilling these expectations. By allowing multiple requests to be handled simultaneously, an asynchronous API can offer a more efficient and responsive service. This is particularly effective for services that require communication between different components or for long-running requests that would otherwise block the server.
The advantage of asynchronous APIs extends beyond just performance. They also enhance the real-time user experience by providing immediate feedback and allowing background processing to continue without disrupting the user’s interaction with the application. Messaging protocols like WebSockets, Kafka, and AMQP offer various options for real-time communication, enabling event-driven interactions that can push notifications to clients as events occur.
In scenarios where connectivity is unreliable or when handling requests that take a long time to execute, asynchronous APIs shine. They are particularly well-suited for applications in sectors like messaging, banking, and gaming, where the speed and efficiency of handling parallel requests can significantly improve user satisfaction and application performance. By implementing asynchronous processing, your API becomes not just a channel for data exchange but a dynamic conduit for real-time engagement and interaction.
Testing and Debugging Your Python REST API
API development is not a journey that ends with deployment, but a continuous cycle of enhancement and improvement. Testing and debugging are the compass and map that guide you through the treacherous terrain of bugs and errors. They are essential practices that ensure the reliability and functionality of your REST API.
Mock services simulate the behavior of an API, providing a sandbox where you can test endpoints and interactions without the need for a fully functioning backend. One particularly useful tool for testing is JSONPlaceholder, which offers a set of fake API endpoints for practicing JSON exchanges. It allows developers to hone their skills in sending and receiving data, all without the overhead of setting up an actual server.
Additionally, the use of Session Objects in testing streamlines the process by maintaining persistent parameters like access tokens across multiple requests. Combining these tools and strategies transforms the testing and debugging process from a daunting task into a manageable and even enjoyable part of development.
By taking the time to thoroughly test and debug your REST API, you not only improve its quality but also build confidence in its stability and reliability. This is an investment that pays dividends in user trust and the overall success of your web service.
Writing Unit Tests for Your API
Unit testing is the act of dissecting your API into its smallest parts and putting each one under the microscope. It’s a meticulous process that involves verifying the behavior of individual components under various conditions. The Python unittest library provides a framework for creating these tests, ensuring that each one is isolated and unaffected by the others. With setUp() and tearDown() methods, you can prepare a clean slate before each test and tidy up afterward, maintaining a controlled environment for your tests to run in.
Using Flask’s app.test_client(), developers can simulate requests to the API and examine the responses without having to run a server. This allows for a granular examination of how the API behaves when faced with different inputs, ensuring that it responds with the correct status codes and data. Basic unit tests may check for successful responses, appropriate data retrieval, and the integrity of the data after various operations.
The true mark of a resilient API is how it handles unexpected or incorrect inputs. Unit tests should account for these scenarios, ensuring that the API behaves predictably and securely even when faced with invalid data. In doing so, you imbue your API with a robustness that is crucial for its long-term viability. Writing comprehensive unit tests is an investment in your API’s future, one that ensures it will continue to serve its purpose reliably and efficiently.
Using Debug Mode and Logging
When it comes to navigating the murky waters of debugging, having a lighthouse in the form of a robust logging system is invaluable. Flask’s debug mode offers an immediate glimpse into the inner workings of your API, providing real-time feedback and error messages that can significantly shorten the debugging process. However, debug mode is not just about catching errors as they happen; it’s also about understanding the flow of your application and recognizing potential issues before they escalate.
The Python logging module, integrated into Flask, allows you to keep a detailed record of events at various severity levels. By setting up basic configuration, you can define which messages are recorded and where they are stored, creating a log that can be invaluable for diagnosing and resolving issues. The format of these log messages can include timestamps, logging level, and custom messages, providing a comprehensive view of your API’s behavior over time.
Armed with a reliable logging system, you can navigate even the most challenging debugging scenarios with confidence. It becomes easier to identify patterns, trace the source of errors, and make informed decisions about how to improve your API. Whether it’s a simple typo or a complex logic error, logging equips you with the tools to uncover the truth and steer your API back on course.
Advanced Topics in Python REST APIs
Delving deeper into the world of REST APIs, you’re bound to come across advanced concepts that can significantly enhance your web services. These topics, such as Hypermedia as the Engine of Application State (HATEOAS) and API versioning, are the fine details that can distinguish a good API from a great one. HATEOAS is an advanced concept that enhances the self-descriptive nature of APIs. Mastering these concepts allows you to build APIs that are not only functional but also adaptable and future-proof.
HATEOAS is an architectural style that extends REST by embedding hypermedia links within the API’s responses. These links guide clients through the available actions based on the current state of the application, simplifying client-side logic and reducing the need for hard-coding URLs. By implementing HATEOAS, you allow your API to self-describe its capabilities, providing a more discoverable and easier-to-use interface for clients.
API versioning is another critical aspect of maintaining a long-lived API. It addresses the challenges of evolving your API without breaking existing clients. Strategies such as URL path versioning, header-based versioning, and even custom media types allow you to introduce changes and new features while maintaining backward compatibility. Properly managing API versions ensures that your API can continue to grow and improve over time, meeting the changing needs of its users without causing disruption.
Hypermedia as the Engine of Application State (HATEOAS)
The concept of HATEOAS is a cornerstone of truly RESTful APIs. It’s a principle that emphasizes the self-descriptive nature of an API, where each response contains not just data but also controls that indicate what can be done next. These hypermedia controls are dynamic and can change depending on the state of the resource, guiding the client through the available options without the need for prior knowledge of the API’s structure.
By embedding links within the API responses, HATEOAS shifts some of the application logic from the client to the server. This means that the client no longer needs to construct URLs or know the specific actions available. Instead, the API provides the relevant links, which the client can simply follow, much like a user browsing through web pages. This reduces the coupling between the client and server, allowing for greater flexibility and easier maintenance.
HATEOAS also aids in informing clients about the current availability of actions. For example, if a particular operation is not currently possible, the corresponding link can be omitted from the response. This helps the client interface to react accordingly, such as by disabling features that are not applicable at the moment. Embedding such intelligence within the API responses makes for a more intuitive and robust user experience, enabling clients to adapt seamlessly to the evolving state of the application.
Managing API Versions
In the ever-evolving landscape of software development, change is the only constant. As your API grows and adapts to new requirements, introducing new versions becomes an inevitable part of the process. When backward-incompatible changes are necessary, rolling out a new version of your API ensures that existing clients are not disrupted. This is where API versioning becomes a critical tool in your arsenal, allowing you to manage and track changes in a controlled and predictable manner.
There are several strategies for versioning an API, each with its own merits:
- URL versioning: incorporating the version number directly into the API’s endpoint paths, making it explicit and easy to manage
- Query parameter and header versioning: offering more subtle approaches, where the version information is included in the HTTP headers or as a query string in the URL
- Consumer-based versioning: tailoring the API’s behavior to the specific needs or preferences of the client.
Regardless of the strategy you choose, adhering to best practices such as semantic versioning can help communicate the nature and scope of changes to your users. Semantic versioning follows a major.minor.patch format, where major changes indicate breaking changes, minor changes add functionality in a backward-compatible manner, and patches are for backward-compatible bug fixes. By clearly communicating these changes and providing a clear deprecation policy, you foster trust and transparency with your API consumers, ensuring a smooth transition as your API evolves.
Summary
As we draw the curtain on this comprehensive guide to mastering Python with REST APIs, we reflect on the journey that has taken us from the fundamental concepts to advanced topics in API development. We have traversed the landscape of RESTful design, learned to set up our Python environment, and delved into the intricacies of creating and securing robust web services. We have also explored performance optimization, testing, and version management, equipping ourselves with the tools to build APIs that are not only functional but also scalable and maintainable.
Throughout this voyage, we’ve seen how Python’s simplicity and readability harmonize with the principles of REST, creating a synergy that makes it an excellent choice for API development. The frameworks and libraries available in the Python ecosystem have served as our allies, simplifying tasks and providing robust solutions for the challenges we’ve faced. By embracing these tools and adhering to best practices, we’ve learned how to craft APIs that stand out for their clarity, security, and responsiveness.
Now, the path ahead is yours to tread. With the knowledge and skills you’ve acquired, you are well-prepared to build your own Python REST APIs, whether for personal projects or at the helm of a team in a professional setting. Remember that the art of API development is one of continuous learning and adaptation. Embrace the challenges and let your creativity flow as you build the web services that could shape the future of the digital world.
Organizations looking for the best tools to support their API management can leverage Moesif’s powerful API analytics and monetization capabilities. Moesif easily integrates with your favorite API management platform or API gateway through one of our easy-to-use plugins, or embed Moesif directly into your API code using one of our SDKs. To try it yourself, sign up today and start with a 14-day free trial; no credit card is required.